最近在学python的内置函数,在此做些笔记,会不断更新的哈
2020年7月28日
abs()函数
这是一个用来求绝对值的函数,返回数值的绝对值
官方介绍:
Return the absolute value of the argument.
print(abs(-1))
输出结果:
1
dict()函数
dict()函数用于创建一个字典
print(dict(city='城市', world='世界'))
print(dict([('city', '城市'), ('world', '世界')]))
print(dict(zip(['city', 'world'], ['城市', '世界'])))
输出结果
{'city': '城市', 'world': '世界'}
{'city': '城市', 'world': '世界'}
{'city': '城市', 'world': '世界'}
help()函数
help()函数是用来获取帮助文档的
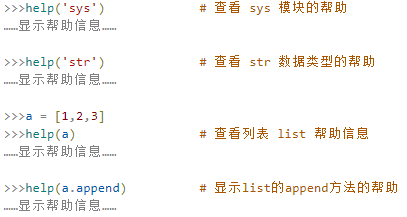
min()函数
求最小值
max()函数
求最大值
all()函数
all() 函数用于判断给定的可迭代参数 iterable 中的所有元素是否都为 TRUE,如果是返回 True,否则返回 False。
元素除了是 0、空、None、False 外都算 True。
参数
- iterable — 元组或列表。
返回值
如果iterable的所有元素不为0、”、False或者iterable为空,all(iterable)返回True,否则返回False;
注意:空元组、空列表、空字典、空集合返回值为True,这里要特别注意。

dir()函数
dir() 函数不带参数时,返回当前范围内的变量、方法和定义的类型列表;
带参数时,返回参数的属性、方法列表。
如果参数包含方法__dir__(),该方法将被调用。如果参数不包含__dir__(),该方法将最大限度地收集参数信息。
给个例子:
print(dir())
输出:
['__annotations__', '__builtins__', '__cached__', '__doc__', '__file__', '__loader__', '__name__', '__package__', '__spec__']
再来一个例子:
print(dir([]))
输出结果:
['add', 'class', 'contains', 'delattr', 'delitem', 'dir', 'doc', 'eq', 'format', 'ge', 'getattribute', 'getitem', 'gt', 'hash', 'iadd', 'imul', 'init', 'init_subclass', 'iter', 'le', 'len', 'lt', 'mul', 'ne', 'new', 'reduce', 'reduce_ex', 'repr', 'reversed', 'rmul', 'setattr', 'setitem', 'sizeof', 'str', 'subclasshook', 'append', 'clear', 'copy', 'count', 'extend', 'index', 'insert', 'pop', 'remove', 'reverse', 'sort']
这样就显示了列表的属性、方法
hex()函数
hex()函数的作用是把十进制数转换为十六进制
例子:
print(hex(15))
print(hex(255))
输出结果:
0xf
0xff
slice()函数
slice()函数用来给序列切片,它有三个参数,分别是
起始索引、终止索引、步长
其中,步长默认为1,可以为负值
举个例子:
list = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
print(list[slice(0, 9, 2)])
print(list[slice(0, 9, 3)])
输出结果如下
[0, 2, 4, 6, 8]
[0, 3, 6]
any()函数
any()函数是用来判断一个可迭代对象是否全为False,如果全为False,则返回False。否则返回True
其中,元素除了是 0、空、FALSE 外都算 TRUE。
举个例子
list = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
print(any(list))
l2 = []
print(any(l2))
输出结果:
True
False
divmod()函数
divmod()函数是一个求整除和余数的函数,返回的是一个元组。包括了整除的结果以及余数
id()函数
id()函数用来返回对象在内存中的地址
object()函数
object()函数用于创建一个对象
举个例子:
a = object()
print(a)
运行结果:
<object object at 0x000001D8CEA67AB0>
sorted()函数
sorted()函数是一个用来排序的函数
sorted(iterable, key=None, reverse=False)
key来自于可迭代对象中
reverse=False即升序,reverse=True即降序
sum()函数
这是一个求和函数,用来求和
ascii()函数
ascii() 函数类似 repr() 函数, 返回一个表示对象的字符串, 但是对于字符串中的非 ASCII 字符则返回通过 repr() 函数使用 \x, \u 或 \U 编码的字符。 生成字符串类似 Python2 版本中 repr() 函数的返回值。
enumerate()函数
enumerate()函数用于将一个可遍历的数据对象(如列表、元组或字符串)组合为一个索引序列,同时列出数据和数据下标,一般用在 for 循环当中。
举个例子
li = ['a', 'b', 'c', 'd', 'e']
print(list(enumerate(li)))
print(list(enumerate(li, start=1)))
for k, v in enumerate(li):
print(k, v)
输出结果:
[(0, 'a'), (1, 'b'), (2, 'c'), (3, 'd'), (4, 'e')]
[(1, 'a'), (2, 'b'), (3, 'c'), (4, 'd'), (5, 'e')]
0 a
1 b
2 c
3 d
4 e
input()函数
input()函数用来从控制台获取内容
举个例子
a = input('input:')
print('Your input is', a)
我们输入123,那么输出结果是
input:123
Your input is 123
oct()函数
oct()函数返回8进制字符串
a = oct(10)
print(a)
print(type(a))
输出结果:
0o12
<class 'str'>
bin()函数
bin()函数返回2进制字符串
a = bin(10)
print(a)
print(type(a))
输出结果
0b1010
<class 'str'>
eval()函数
eval()函数用来执行字符串表达式
a = 7
print(eval('a + 2'))
运行结果
9
int()函数
int()函数用于将一个数字或字符串转换为整型
print(int('0xa', 16))
print(int('1001', 2))
print(int(3.6))
输出结果
10
9
3
bool()函数
bool()函数用于将给定参数转换为bool类型。若没有给定参数,则返回False
print(bool(0))
print(bool(-1))
print(bool(1))
print(bool(2))
输出结果:
False
True
True
True
exec()函数
exec 执行储存在字符串或文件中的 Python 语句,相比于 eval,exec可以执行更复杂的 Python 代码。
举个例子
exec("print('hello')")
exec("""for i in range(5):
print ("iter time: %d" % i)
""")
输出结果
hello
iter time: 0
iter time: 1
iter time: 2
iter time: 3
iter time: 4
isinstance()函数
isinstance()函数用于判断对象是否为同一类型,返回True或False
a = 2
print(isinstance(a, int))
print(isinstance(a, str))
print(isinstance(a, (str, int, tuple)))#是元组中的一个则返回True
输出结果
True
False
True
isinstance() 与 type() 区别:
- type() 不会认为子类是一种父类类型,不考虑继承关系。
- isinstance() 会认为子类是一种父类类型,考虑继承关系。
如果要判断两个类型是否相同推荐使用 isinstance()。
class A:
pass
class B(A):
pass
isinstance(A(), A) # returns True
type(A()) == A # returns True
isinstance(B(), A) # returns True
type(B()) == A # returns False
ord()函数
ord()函数是 chr() 函数(对于 8 位的 ASCII 字符串)的配对函数,它以一个字符串(Unicode 字符)作为参数,返回对应的 ASCII 数值,或者 Unicode 数值。
举个例子
print(ord('8'))
print(ord('9'))
print(ord('帅'))
输出结果
56
57
24069
bytearray()函数
bytearray() 方法返回一个新字节数组。这个数组里的元素是可变的,并且每个元素的值范围: 0 <= x < 256。
print(bytearray([1,2,3]))
print(bytearray('longjin666', 'utf-8'))
str()函数
把一个对象转换为字符串
pow()函数
pow() 方法返回 xy(x的y次方) 的值。
pow()函数有两种调用方式,一种是math.pow(x, y),另一种是内置函数pow(x, y)
pow() 通过内置的方法直接调用,内置方法会把参数作为整型,而 math 模块则会把参数转换为 float。
举个例子:
import math
print(pow(2, 3))
print(math.pow(2, 3))
输出结果:
8
8.0
zip()函数
zip() 函数用于将可迭代的对象作为参数,将对象中对应的元素打包成一个个元组,然后返回由这些元组组成的对象,这样做的好处是节约了不少的内存。
我们可以使用 list() 转换来输出列表。
如果各个迭代器的元素个数不一致,则返回列表长度与最短的对象相同,利用 * 号操作符,可以将元组解压为列表。
a = [1, 2, 3]
b = [4, 5, 6]
zipped = zip(a, b)
print(zipped)
print(list(zipped))
x, y = zip(*zip(b, a))#解压缩,展开为二维矩阵
print(list(x))
print(list(y))
输出结果
<zip object at 0x00000190A712E588>
[(1, 4), (2, 5), (3, 6)]
[4, 5, 6]
[1, 2, 3]
set()函数
set()函数用来创建集合
a = set() #创建空集
a = set('ShiMen Middle School')
print(a)
输出结果
{'e', 'o', 'S', 'M', 'n', 'i', ' ', 'd', 'l', 'h', 'c'}
round()函数
round()函数用来四舍五入(准确来说是5以上才“入”,因为round(4.5)的结果是4
print(round(4.5145415))
print(round(3.1415926535, 2))
print(round(3.1415926535, 3))
print(round(3.1415926535, 4))
print(round(3.1415926535, 5))
输出结果
5
3.14
3.142
3.1416
3.14159